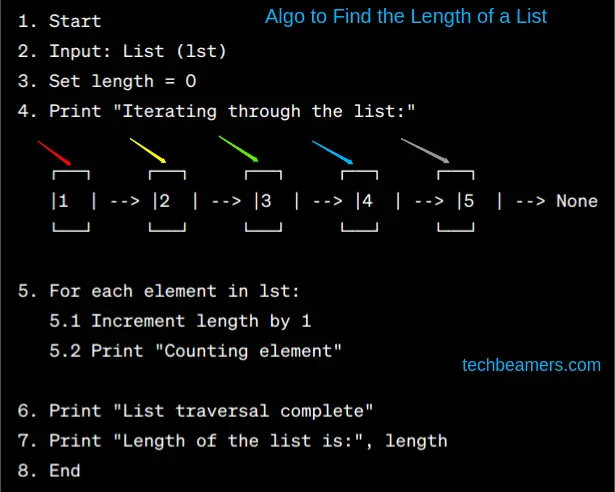
In Python, lists are one of the most versatile and commonly used data structures. Whether you’re working with a small dataset or large-scale data processing, knowing how to determine the size or length of a list is fundamental to many programming tasks. This basic operation plays a critical role in everything from iterating over elements to performing calculations or managing dynamic data. In this article, we explore why understanding the length of a list matters, common scenarios where you need to know list size, and why this knowledge is essential for effective Python programming.
The Importance of Knowing the Length of a List
Effective Data Management
In Python, lists are often used to store collections of data, making it essential to know how many items are within the list. Whether you’re organizing user input, storing processed data, or managing complex data structures, understanding the list size allows you to perform critical operations with accuracy. Operations like sorting, filtering, and segmenting data depend on knowing how many elements are in the list before proceeding. For instance, when manipulating data dynamically, the list size dictates how the program handles the data as it grows or shrinks.
Proper Iteration in Loops
When working with lists, loops are frequently employed to access or manipulate each element. However, knowing the length of a list beforehand ensures that you can properly structure your loops. This is especially important when dealing with large datasets. By determining the length of the list, you can control the range of your loop and avoid errors, such as accessing an element at an index that does not exist, which can lead to runtime errors.
Handling Dynamic Data
In many real-world applications, the size of a list can change dynamically as elements are added or removed. For example, in data processing applications, new data might be appended to a list, or irrelevant data might be removed. Keeping track of the length of the list at any given time ensures your program adapts correctly to these changes. Without knowing the length, you risk incorrect data processing or errors in data handling.
Preventing Errors
An understanding of the list’s size also helps in preventing common programming errors. One of the most frequent errors in Python when dealing with lists is an IndexError, which occurs when you attempt to access an element at an index that exceeds the list’s length. By knowing the exact number of items in your list, you can prevent such errors and make your code more reliable.
The Versatility of Python Lists
Python lists are widely used because of their flexibility. A list can hold any type of data, including integers, strings, and even other lists. This makes lists a powerful tool for a variety of programming tasks, from basic data handling to complex algorithms.
While the data types of the elements within the list can vary, one thing remains constant: the need to determine the list’s length. Whether you’re processing a list of numbers, handling strings, or working with mixed data types, knowing the size of the list is crucial for accurate and efficient data manipulation.
Key Scenarios for Checking List Size
Validating Data Before Operations
Before performing operations on a list, it’s often necessary to check if the list contains the expected number of elements. This is particularly important when you’re performing data validation or pre-processing. For example, if you expect a list to contain at least ten elements before you begin analyzing it, checking the list size allows you to avoid errors that would arise from trying to process incomplete data.
Loops and Conditional Logic
Many Python applications rely on loops to iterate over data stored in lists. By knowing the length of the list, you can define the exact number of iterations required for efficient data processing. This is especially beneficial when you’re dealing with lists that grow dynamically, ensuring that your loops remain accurate and don’t run into errors when the data changes.
For example, if you are using a loop to search for specific elements within a list, knowing the size allows you to set up the loop properly, ensuring that it covers all possible indices and doesn’t attempt to access invalid ones.
Resizing and Modifying Lists Dynamically
Some Python programs involve modifying lists dynamically. Whether you are appending new data, removing elements, or reordering items, knowing the list’s size allows you to control these changes better. When resizing lists, checking the size of the list is critical to understanding how to proceed. For instance, when deciding whether to add new elements or truncate the list, knowing how many elements are currently in the list can inform your decisions.
Applying Logic Based on List Size
Another reason to check the size of a list is to apply specific logic or behavior based on the list’s length. In many cases, the actions you take depend on the number of elements present. For example, if you are writing a function that processes user input, you might want to perform one type of operation if the list contains fewer than five items and a different operation if the list has more than five.
Best Practices for List Size Management
Efficient Data Handling
In Python, managing lists efficiently means knowing their size at the right time. For example, when processing large datasets, determining the list size upfront allows you to allocate resources better and avoid excessive memory usage or unnecessary calculations. By knowing the size, you can optimize the way you iterate through the list and how you manage the data as it grows.
Avoiding Redundant Operations
When working with multiple lists or complex data structures, avoiding redundant operations is crucial. Knowing the size of each list allows you to prevent unnecessary iterations or operations that could slow down your program. This is especially true when dealing with multiple lists, as redundant checks or iterations could waste time and resources.
Writing Robust Code
Knowing the length of a list also contributes to writing more robust code. By checking the size of the list, you can ensure that your program only attempts operations that are feasible based on the available data. For instance, if you’re working with a user-submitted list, confirming the length can help you avoid bugs related to data inconsistency or incomplete input.
Conclusion
Understanding how to determine the length of a list is an essential skill for any Python developer. Whether you’re handling small data sets or large-scale data processing tasks, knowing the size of a list is critical for managing data effectively, ensuring efficient program execution, and preventing errors. By incorporating list size checks into your Python programs, you enhance your coding practices and ensure that your applications remain scalable and error-free.
Whether you’re working with static or dynamic data, handling complex algorithms, or simply iterating over a list, the ability to determine its size will improve your program’s performance and reliability. By mastering this concept, you’ll be better equipped to tackle a wide range of Python programming challenges with confidence and precision.